basic approach is to change connection node.next = node.next.next
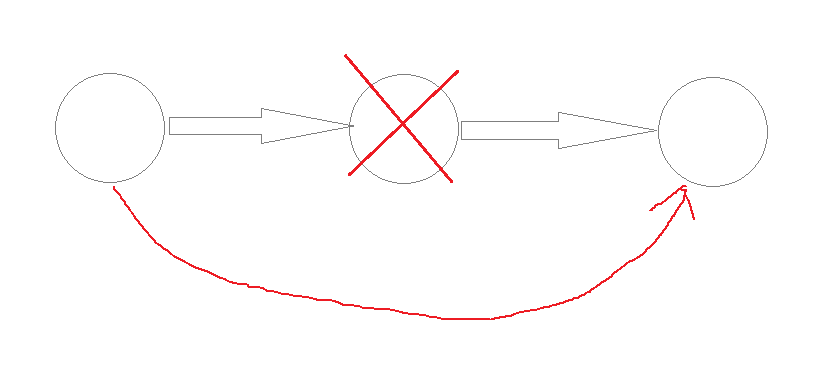
1. RemoveElements(ListNode head, int val)
leetcode task
optimal solution through sentinel or fake node
/** * Definition for singly-linked list. * public class ListNode { * int val; * ListNode next; * ListNode() {} * ListNode(int val) { this.val = val; } * ListNode(int val, ListNode next) { this.val = val; this.next = next; } * } */ class Solution { public ListNode removeElements(ListNode head, int val) { //degenerate case if (head == null) return null; //creating additional node to the left from first and a variable "sentinel" with reference to it ListNode node; ListNode sentinel = new ListNode(); sentinel.next = head; node = sentinel; //checking node value and deleting reference to it if conditions are met while (node.next.next != null){ if (node.next.val == val){ node.next = node.next.next; continue; } node = node.next; } //edge case (last ListNode element) if (node.next.val == val) node.next = null; return sentinel.next; } }
not optimal solution with border cases
/** * Definition for singly-linked list. * public class ListNode { * public int val; * public ListNode next; * public ListNode(int val=0, ListNode next=null) { * this.val = val; * this.next = next; * } * } */ public class Solution { public ListNode RemoveElements(ListNode head, int val) { if (head == null) return null; ListNode res = head; ListNode current = head; ListNode previous = null; // bool wasDeletedFromMiddle = false; while (current != null) { if (current.val == val) { if (current == head) { head = head.next; res = head; } else if (current != head && current.next != null) { if (current.next != null && current.next.val == val) { current = current.next; continue; } previous.next = current.next; } // tail else if (current != head && current.next == null) { previous.next = null; } } previous = current; current = current.next; } return res; } }
2. DeleteNode(ListNode node)
leetcode
Here we don’t have access to previos node

public void DeleteNode(ListNode node) {
node.val = node.next.val;
node.next = node.next.next;
}